#include <rapidxml.hpp>
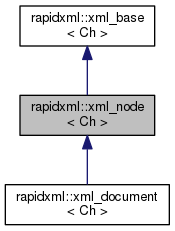
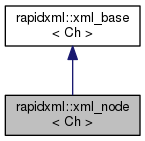
Public Member Functions | |
xml_node (node_type type) | |
node_type | type () const |
xml_document< Ch > * | document () const |
xml_node< Ch > * | first_node (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_node< Ch > * | last_node (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_node< Ch > * | previous_sibling (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_node< Ch > * | next_sibling (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_attribute< Ch > * | first_attribute (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_attribute< Ch > * | last_attribute (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
void | type (node_type type) |
void | prepend_node (xml_node< Ch > *child) |
void | append_node (xml_node< Ch > *child) |
void | insert_node (xml_node< Ch > *where, xml_node< Ch > *child) |
void | remove_first_node () |
void | remove_last_node () |
void | remove_node (xml_node< Ch > *where) |
Removes specified child from the node. | |
void | remove_all_nodes () |
Removes all child nodes (but not attributes). | |
void | prepend_attribute (xml_attribute< Ch > *attribute) |
void | append_attribute (xml_attribute< Ch > *attribute) |
void | insert_attribute (xml_attribute< Ch > *where, xml_attribute< Ch > *attribute) |
void | remove_first_attribute () |
void | remove_last_attribute () |
void | remove_attribute (xml_attribute< Ch > *where) |
void | remove_all_attributes () |
Removes all attributes of node. | |
![]() | |
Ch * | name () const |
std::size_t | name_size () const |
Ch * | value () const |
std::size_t | value_size () const |
void | name (const Ch *name, std::size_t size) |
void | name (const Ch *name) |
void | value (const Ch *value, std::size_t size) |
void | value (const Ch *value) |
xml_node< Ch > * | parent () const |
Additional Inherited Members | |
![]() | |
static Ch * | nullstr () |
![]() | |
Ch * | m_name |
Ch * | m_value |
std::size_t | m_name_size |
std::size_t | m_value_size |
xml_node< Ch > * | m_parent |
Detailed Description
template<class Ch = char>
class rapidxml::xml_node< Ch >
Class representing a node of XML document. Each node may have associated name and value strings, which are available through name() and value() functions. Interpretation of name and value depends on type of the node. Type of node can be determined by using type() function.
Note that after parse, both name and value of node, if any, will point interior of source text used for parsing. Thus, this text must persist in the memory for the lifetime of node.
- Parameters
-
Ch Character type to use.
Constructor & Destructor Documentation
|
inline |
Constructs an empty node with the specified type. Consider using memory_pool of appropriate document to allocate nodes manually.
- Parameters
-
type Type of node to construct.
Member Function Documentation
|
inline |
Appends a new attribute to the node.
- Parameters
-
attribute Attribute to append.
|
inline |
Appends a new child node. The appended child becomes the last child.
- Parameters
-
child Node to append.
|
inline |
Gets document of which node is a child.
- Returns
- Pointer to document that contains this node, or 0 if there is no parent document.
|
inline |
Gets first attribute of node, optionally matching attribute name.
- Parameters
-
name Name of attribute to find, or 0 to return first attribute regardless of its name; this string doesn't have to be zero-terminated if name_size is non-zero name_size Size of name, in characters, or 0 to have size calculated automatically from string case_sensitive Should name comparison be case-sensitive; non case-sensitive comparison works properly only for ASCII characters
- Returns
- Pointer to found attribute, or 0 if not found.
|
inline |
Gets first child node, optionally matching node name.
- Parameters
-
name Name of child to find, or 0 to return first child regardless of its name; this string doesn't have to be zero-terminated if name_size is non-zero name_size Size of name, in characters, or 0 to have size calculated automatically from string case_sensitive Should name comparison be case-sensitive; non case-sensitive comparison works properly only for ASCII characters
- Returns
- Pointer to found child, or 0 if not found.
|
inline |
Inserts a new attribute at specified place inside the node. All attributes after and including the specified attribute are moved one position back.
- Parameters
-
where Place where to insert the attribute, or 0 to insert at the back. attribute Attribute to insert.
|
inline |
Inserts a new child node at specified place inside the node. All children after and including the specified node are moved one position back.
- Parameters
-
where Place where to insert the child, or 0 to insert at the back. child Node to insert.
|
inline |
Gets last attribute of node, optionally matching attribute name.
- Parameters
-
name Name of attribute to find, or 0 to return last attribute regardless of its name; this string doesn't have to be zero-terminated if name_size is non-zero name_size Size of name, in characters, or 0 to have size calculated automatically from string case_sensitive Should name comparison be case-sensitive; non case-sensitive comparison works properly only for ASCII characters
- Returns
- Pointer to found attribute, or 0 if not found.
|
inline |
Gets last child node, optionally matching node name. Behaviour is undefined if node has no children. Use first_node() to test if node has children.
- Parameters
-
name Name of child to find, or 0 to return last child regardless of its name; this string doesn't have to be zero-terminated if name_size is non-zero name_size Size of name, in characters, or 0 to have size calculated automatically from string case_sensitive Should name comparison be case-sensitive; non case-sensitive comparison works properly only for ASCII characters
- Returns
- Pointer to found child, or 0 if not found.
|
inline |
Gets next sibling node, optionally matching node name. Behaviour is undefined if node has no parent. Use parent() to test if node has a parent.
- Parameters
-
name Name of sibling to find, or 0 to return next sibling regardless of its name; this string doesn't have to be zero-terminated if name_size is non-zero name_size Size of name, in characters, or 0 to have size calculated automatically from string case_sensitive Should name comparison be case-sensitive; non case-sensitive comparison works properly only for ASCII characters
- Returns
- Pointer to found sibling, or 0 if not found.
|
inline |
Prepends a new attribute to the node.
- Parameters
-
attribute Attribute to prepend.
|
inline |
Prepends a new child node. The prepended child becomes the first child, and all existing children are moved one position back.
- Parameters
-
child Node to prepend.
|
inline |
Gets previous sibling node, optionally matching node name. Behaviour is undefined if node has no parent. Use parent() to test if node has a parent.
- Parameters
-
name Name of sibling to find, or 0 to return previous sibling regardless of its name; this string doesn't have to be zero-terminated if name_size is non-zero name_size Size of name, in characters, or 0 to have size calculated automatically from string case_sensitive Should name comparison be case-sensitive; non case-sensitive comparison works properly only for ASCII characters
- Returns
- Pointer to found sibling, or 0 if not found.
|
inline |
Removes specified attribute from node.
- Parameters
-
where Pointer to attribute to be removed.
|
inline |
Removes first attribute of the node. If node has no attributes, behaviour is undefined. Use first_attribute() to test if node has attributes.
|
inline |
Removes first child node. If node has no children, behaviour is undefined. Use first_node() to test if node has children.
|
inline |
Removes last attribute of the node. If node has no attributes, behaviour is undefined. Use first_attribute() to test if node has attributes.
|
inline |
Removes last child of the node. If node has no children, behaviour is undefined. Use first_node() to test if node has children.
|
inline |
Gets type of node.
- Returns
- Type of node.
|
inline |
Sets type of node.
- Parameters
-
type Type of node to set.
The documentation for this class was generated from the following file:
- src/forsyde/rapidxml.hpp
Generated by Doxygen 1.8.11