#include <rapidxml.hpp>
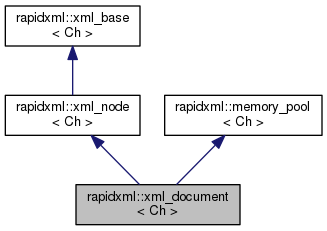
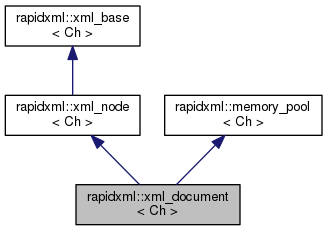
Public Member Functions | |
xml_document () | |
Constructs empty XML document. | |
template<int Flags> | |
void | parse (Ch *text) |
void | clear () |
![]() | |
xml_node (node_type type) | |
node_type | type () const |
xml_document< Ch > * | document () const |
xml_node< Ch > * | first_node (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_node< Ch > * | last_node (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_node< Ch > * | previous_sibling (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_node< Ch > * | next_sibling (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_attribute< Ch > * | first_attribute (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
xml_attribute< Ch > * | last_attribute (const Ch *name=0, std::size_t name_size=0, bool case_sensitive=true) const |
void | type (node_type type) |
void | prepend_node (xml_node< Ch > *child) |
void | append_node (xml_node< Ch > *child) |
void | insert_node (xml_node< Ch > *where, xml_node< Ch > *child) |
void | remove_first_node () |
void | remove_last_node () |
void | remove_node (xml_node< Ch > *where) |
Removes specified child from the node. | |
void | remove_all_nodes () |
Removes all child nodes (but not attributes). | |
void | prepend_attribute (xml_attribute< Ch > *attribute) |
void | append_attribute (xml_attribute< Ch > *attribute) |
void | insert_attribute (xml_attribute< Ch > *where, xml_attribute< Ch > *attribute) |
void | remove_first_attribute () |
void | remove_last_attribute () |
void | remove_attribute (xml_attribute< Ch > *where) |
void | remove_all_attributes () |
Removes all attributes of node. | |
![]() | |
Ch * | name () const |
std::size_t | name_size () const |
Ch * | value () const |
std::size_t | value_size () const |
void | name (const Ch *name, std::size_t size) |
void | name (const Ch *name) |
void | value (const Ch *value, std::size_t size) |
void | value (const Ch *value) |
xml_node< Ch > * | parent () const |
![]() | |
memory_pool () | |
Constructs empty pool with default allocator functions. | |
~memory_pool () | |
xml_node< Ch > * | allocate_node (node_type type, const Ch *name=0, const Ch *value=0, std::size_t name_size=0, std::size_t value_size=0) |
xml_attribute< Ch > * | allocate_attribute (const Ch *name=0, const Ch *value=0, std::size_t name_size=0, std::size_t value_size=0) |
Ch * | allocate_string (const Ch *source=0, std::size_t size=0) |
xml_node< Ch > * | clone_node (const xml_node< Ch > *source, xml_node< Ch > *result=0) |
void | clear () |
void | set_allocator (alloc_func *af, free_func *ff) |
Additional Inherited Members | |
![]() | |
static Ch * | nullstr () |
![]() | |
Ch * | m_name |
Ch * | m_value |
std::size_t | m_name_size |
std::size_t | m_value_size |
xml_node< Ch > * | m_parent |
Detailed Description
template<class Ch = char>
class rapidxml::xml_document< Ch >
This class represents root of the DOM hierarchy. It is also an xml_node and a memory_pool through public inheritance. Use parse() function to build a DOM tree from a zero-terminated XML text string. parse() function allocates memory for nodes and attributes by using functions of xml_document, which are inherited from memory_pool. To access root node of the document, use the document itself, as if it was an xml_node.
- Parameters
-
Ch Character type to use.
Member Function Documentation
|
inline |
Clears the document by deleting all nodes and clearing the memory pool. All nodes owned by document pool are destroyed.
|
inline |
Parses zero-terminated XML string according to given flags. Passed string will be modified by the parser, unless rapidxml::parse_non_destructive flag is used. The string must persist for the lifetime of the document. In case of error, rapidxml::parse_error exception will be thrown.
If you want to parse contents of a file, you must first load the file into the memory, and pass pointer to its beginning. Make sure that data is zero-terminated.
Document can be parsed into multiple times. Each new call to parse removes previous nodes and attributes (if any), but does not clear memory pool.
- Parameters
-
text XML data to parse; pointer is non-const to denote fact that this data may be modified by the parser.
The documentation for this class was generated from the following file:
- src/forsyde/rapidxml.hpp
Generated by Doxygen 1.8.11