sy_helpers.hpp File Reference
Implements helper primitives for modeling in the SY MoC. More...
#include <functional>
#include <tuple>
#include "abst_ext.hpp"
#include "sy_process_constructors.hpp"
Include dependency graph for sy_helpers.hpp:
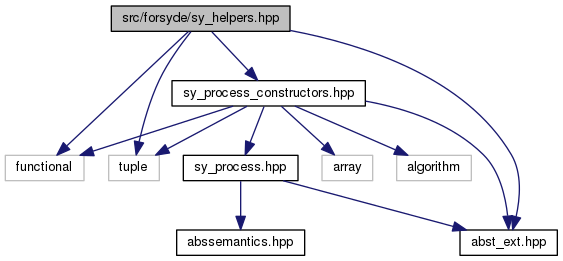
This graph shows which files directly or indirectly include this file:
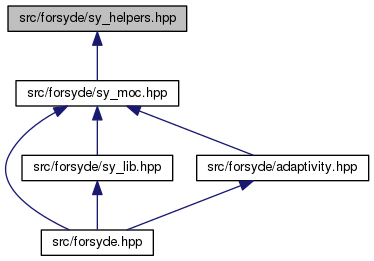
Go to the source code of this file.
Namespaces | |
ForSyDe | |
The namespace for ForSyDe. | |
ForSyDe::SY | |
The namespace for synchronous MoC. | |
Functions | |
template<class T0 , template< class > class OIf, class T1 , template< class > class I1If> | |
comb< T0, T1 > * | ForSyDe::SY::make_comb (const std::string &pName, const typename comb< T0, T1 >::functype &_func, OIf< T0 > &outS, I1If< T1 > &inp1S) |
Helper function to construct a comb process. More... | |
template<class T0 , template< class > class OIf, class T1 , template< class > class I1If, class T2 , template< class > class I2If> | |
comb2< T0, T1, T2 > * | ForSyDe::SY::make_comb2 (const std::string &pName, const typename comb2< T0, T1, T2 >::functype &_func, OIf< T0 > &outS, I1If< T1 > &inp1S, I2If< T2 > &inp2S) |
Helper function to construct a comb2 process. More... | |
template<class T0 , template< class > class OIf, class T1 , template< class > class I1If, class T2 , template< class > class I2If, class T3 , template< class > class I3If> | |
comb3< T0, T1, T2, T3 > * | ForSyDe::SY::make_comb3 (const std::string &pName, const typename comb3< T0, T1, T2, T3 >::functype &_func, OIf< T0 > &outS, I1If< T1 > &inp1S, I2If< T2 > &inp2S, I3If< T3 > &inp3S) |
Helper function to construct a comb3 process. More... | |
template<class T0 , template< class > class OIf, class T1 , template< class > class I1If, class T2 , template< class > class I2If, class T3 , template< class > class I3If, class T4 , template< class > class I4If> | |
comb4< T0, T1, T2, T3, T4 > * | ForSyDe::SY::make_comb4 (const std::string &pName, const typename comb4< T0, T1, T2, T3, T4 >::functype &_func, OIf< T0 > &outS, I1If< T1 > &inp1S, I2If< T2 > &inp2S, I3If< T3 > &inp3S, I4If< T4 > &inp4S) |
Helper function to construct a comb4 process. More... | |
template<class T0 , template< class > class OIf, class T1 , template< class > class IIf, std::size_t N> | |
combX< T0, T1, N > * | ForSyDe::SY::make_combX (const std::string &pName, const typename combX< T0, T1, N >::functype &_func, OIf< T0 > &outS, std::array< IIf< T1 >, N > &inpS) |
Helper function to construct a combX process. More... | |
template<typename T , template< class > class IIf, template< class > class OIf> | |
delay< T > * | ForSyDe::SY::make_delay (const std::string &pName, const abst_ext< T > &initval, OIf< T > &outS, IIf< T > &inpS) |
Helper function to construct a delay process. More... | |
template<typename T , template< class > class IIf, template< class > class OIf> | |
delayn< T > * | ForSyDe::SY::make_delayn (const std::string &pName, const abst_ext< T > &initval, const unsigned int &n, OIf< T > &outS, IIf< T > &inpS) |
Helper function to construct a delayn process. More... | |
template<typename IT , typename ST , typename OT , template< class > class IIf, template< class > class OIf> | |
moore< IT, ST, OT > * | ForSyDe::SY::make_moore (const std::string &pName, const typename moore< IT, ST, OT >::ns_functype &_ns_func, const typename moore< IT, ST, OT >::od_functype &_od_func, const ST &init_st, OIf< OT > &outS, IIf< IT > &inpS) |
Helper function to construct a moore process. More... | |
template<typename IT , typename ST , typename OT , template< class > class IIf, template< class > class OIf> | |
mealy< IT, ST, OT > * | ForSyDe::SY::make_mealy (const std::string &pName, const typename mealy< IT, ST, OT >::ns_functype &_ns_func, const typename mealy< IT, ST, OT >::od_functype &_od_func, const ST &init_st, OIf< OT > &outS, IIf< IT > &inpS) |
Helper function to construct a mealy process. More... | |
template<typename T , template< class > class IIf, template< class > class OIf> | |
fill< T > * | ForSyDe::SY::make_fill (const std::string &pName, const T &def_val, OIf< T > &outS, IIf< T > &inpS) |
Helper function to construct a fill process. More... | |
template<typename T , template< class > class IIf, template< class > class OIf> | |
hold< T > * | ForSyDe::SY::make_hold (const std::string &pName, const T &def_val, OIf< T > &outS, IIf< T > &inpS) |
Helper function to construct a hold process. More... | |
template<typename T , template< class > class IIf, template< class > class OIf> | |
group< T > * | ForSyDe::SY::make_group (const std::string &pName, const unsigned long &samples, OIf< std::vector< abst_ext< T >>> &outS, IIf< T > &inpS) |
Helper function to construct a group process. More... | |
template<class T , template< class > class OIf> | |
constant< T > * | ForSyDe::SY::make_constant (const std::string &pName, const abst_ext< T > &initval, const unsigned long long &take, OIf< T > &outS) |
Helper function to construct a constant source process. More... | |
template<class T , template< class > class OIf> | |
source< T > * | ForSyDe::SY::make_source (const std::string &pName, const typename source< T >::functype &_func, const abst_ext< T > &initval, const unsigned long long &take, OIf< T > &outS) |
Helper function to construct a source process. More... | |
template<class T , template< class > class OIf> | |
file_source< T > * | ForSyDe::SY::make_file_source (std::string pName, typename file_source< T >::functype _func, std::string file_name, OIf< T > &outS) |
Helper function to construct a file_source process. More... | |
template<class T , template< class > class OIf> | |
vsource< T > * | ForSyDe::SY::make_vsource (const std::string &pName, const std::vector< abst_ext< T >> &in_vec, OIf< T > &outS) |
Helper function to construct a vector source process. More... | |
template<class T , template< class > class IIf> | |
sink< T > * | ForSyDe::SY::make_sink (const std::string &pName, const typename sink< T >::functype &_func, IIf< T > &inS) |
Helper function to construct a sink process. More... | |
template<class T , template< class > class IIf> | |
file_sink< T > * | ForSyDe::SY::make_file_sink (std::string pName, typename file_sink< T >::functype _func, std::string file_name, IIf< T > &inS) |
Helper function to construct a file_sink process. More... | |
template<class T1 , template< class > class I1If, class T2 , template< class > class I2If, template< class > class OIf> | |
zip< T1, T2 > * | ForSyDe::SY::make_zip (const std::string &pName, OIf< std::tuple< abst_ext< T1 >, abst_ext< T2 >>> &outS, I1If< T1 > &inp1S, I2If< T2 > &inp2S) |
Helper function to construct a zip process. More... | |
template<class T1 , std::size_t N, template< class > class OIf> | |
zipX< T1, N > * | ForSyDe::SY::make_zipX (const std::string &pName, OIf< std::array< abst_ext< T1 >, N >> &outS) |
Helper function to construct a zipX process. More... | |
template<template< class > class IIf, class T1 , template< class > class O1If, class T2 , template< class > class O2If> | |
unzip< T1, T2 > * | ForSyDe::SY::make_unzip (const std::string &pName, IIf< std::tuple< abst_ext< T1 >, abst_ext< T2 >>> &inpS, O1If< T1 > &out1S, O2If< T2 > &out2S) |
Helper function to construct an unzip process. More... | |
template<template< class > class IIf, class T1 , std::size_t N> | |
unzipX< T1, N > * | ForSyDe::SY::make_unzipX (const std::string &pName, IIf< std::array< abst_ext< T1 >, N >> &inpS) |
Helper function to construct an unzipX process. More... | |
template<typename T , template< class > class IIf, template< class > class OIf> | |
fanout< T > * | ForSyDe::SY::make_fanout (const std::string &pName, OIf< T > &outS, IIf< T > &inpS) |
Helper function to construct a fanout process. More... | |
Detailed Description
Implements helper primitives for modeling in the SY MoC.
This file includes helper functions which facilliate construction of processes in the SY MoC
Generated by Doxygen 1.8.11